Key Takeaways
- Importance of securing PHP web applications against modern cyber threats
- Best practices for PHP code security
- Implementing secure authentication and authorization techniques
- Strategies for protecting data and user privacy in PHP applications
- Understanding and mitigating common PHP security vulnerabilities
In the ever-evolving world of web development, security remains a paramount concern, especially for applications built with PHP. This popular server-side scripting language powers a significant portion of the web, making PHP applications a frequent target for cyber-attacks. Understanding how to fortify your PHP web applications against threats is crucial in safeguarding your data and maintaining user trust.
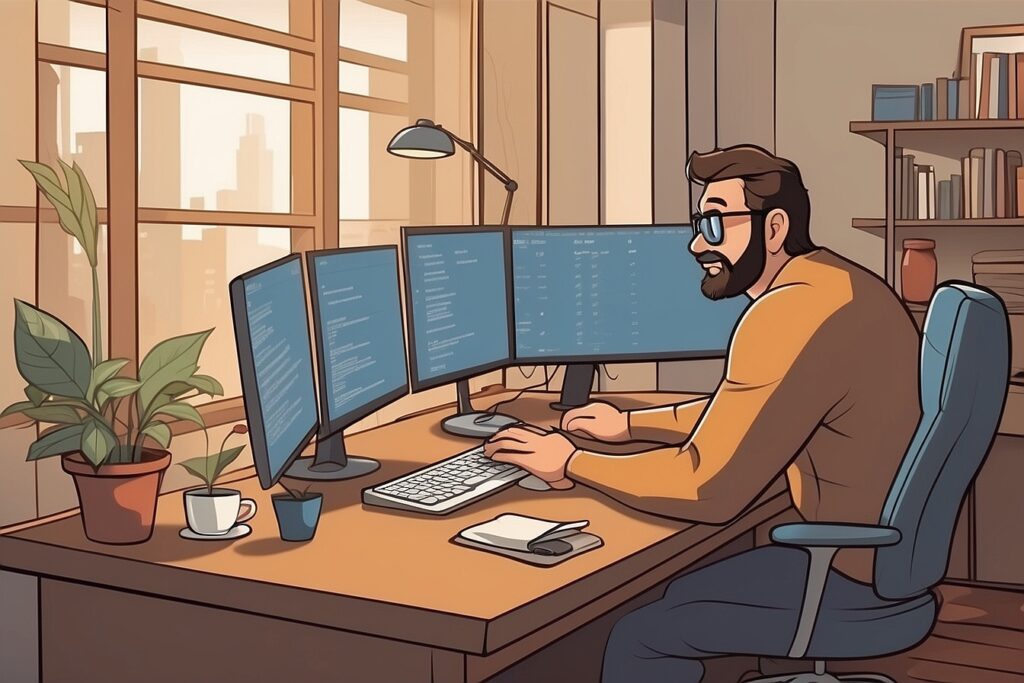
PHP Security Fundamentals
Securing a PHP web application starts with a solid foundation in security principles. This involves understanding the nature of web threats and how they can affect PHP applications. Common threats include SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF). Developers should be well-versed in these concepts and how they manifest in the PHP environment. It’s essential to stay updated on the latest PHP versions, as they often include security enhancements and vulnerability patches. Regularly updating your PHP version can prevent many common security issues.
Secure Coding Practices
PHP developers must adopt secure coding practices to minimize vulnerabilities. This involves writing code that anticipates and mitigates potential security threats. For example, when dealing with user input, it’s crucial to validate and sanitize data to prevent SQL injection attacks. Using prepared statements with PDO (PHP Data Objects) or MySQLi can significantly reduce these risks. Furthermore, error handling should be managed carefully to avoid revealing sensitive information. Displaying detailed error messages to users can expose your application’s internal structure, making it more susceptible to attacks.
Implementing Authentication and Authorization
Authentication and authorization are critical components of web application security. For PHP applications, this means ensuring that users are who they claim to be (authentication) and have permission to access certain resources (authorization). Implementing secure login mechanisms, such as two-factor authentication, can greatly enhance security. Passwords should be stored securely using hashing algorithms like bcrypt. PHP’s password_hash()
and password_verify()
functions provide a robust and straightforward way to handle password security.
Session and Cookie Security
Sessions and cookies are integral to maintaining state and user data across multiple page requests. However, they can be exploited if not handled securely. Developers should use secure session management techniques, like regenerating session IDs after login and ensuring cookies are set with the Secure
and HttpOnly
flags. This prevents session hijacking and cookie theft. Additionally, consider implementing timeouts for sessions to reduce the risk of unauthorized access.
Data Encryption and Protection
Protecting sensitive data, both in transit and at rest, is a cornerstone of PHP application security. Data in transit should be encrypted using SSL/TLS, ensuring all communication between the client and server is secure. This prevents man-in-the-middle attacks and eavesdropping. For data at rest, such as in databases, encryption should be employed to safeguard against data breaches. PHP provides functions like openssl_encrypt()
and openssl_decrypt()
for robust data encryption.
Preventing Cross-Site Scripting (XSS) in PHP
Cross-Site Scripting (XSS) is a common attack where malicious scripts are injected into web pages viewed by other users. In PHP, preventing XSS involves careful handling of user input and output encoding. To mitigate XSS risks:
Content Security Policy (CSP)
Implementing a Content Security Policy (CSP) is an effective defense against XSS. CSP allows you to specify which resources can be loaded and executed by the browser. By setting appropriate directives in your CSP header, you can prevent the browser from executing unauthorized scripts, even if they are injected into your page.
Output Encoding
Always encode output data before rendering it to the user. PHP offers functions like htmlspecialchars()
and htmlentities()
to encode special characters, which can prevent script injection. For example, converting characters like <
, >
, and &
to their HTML entities ensures that user-supplied data is treated as plain text, not executable code.
Validating and Sanitizing Input
Validate all user inputs to ensure they meet your criteria (e.g., type, format, length). Sanitize inputs by removing or encoding potentially harmful characters. PHP’s filter functions, like filter_var()
, can be used for this purpose, providing a variety of filters and flags for different data types.
Mitigating Cross-Site Request Forgery (CSRF) in PHP
Cross-Site Request Forgery (CSRF) occurs when a malicious website causes a user’s browser to perform an unwanted action on another site where the user is authenticated. To combat CSRF:
Anti-CSRF Tokens
Use anti-CSRF tokens in forms. These tokens are random strings, unique to each session and form. The token is verified on the server-side when the form is submitted, ensuring the request originated from your application.
SameSite Cookie Attribute
Set the SameSite attribute for cookies. This attribute controls whether a cookie is sent with cross-site requests. Setting it to Strict
or Lax
can prevent your cookies from being sent in requests initiated by third-party websites, reducing the risk of CSRF attacks.
Database Security in PHP Applications
Secure interaction with databases is crucial to prevent attacks like SQL injection. In addition to using prepared statements, consider the following:
Least Privilege Principle
When connecting to a database, use an account with the minimum necessary permissions. Avoid using the root or admin account, which can access and modify all data and database settings.
Regular Audits and Backups
Regularly audit your database for unusual activities or unauthorized changes. Implement a robust backup strategy to recover data in case of breaches or accidental data loss.
Secure File Handling in PHP
File handling, particularly when dealing with user uploads, can be a vulnerability if not handled properly:
Validating File Uploads
Validate file types and sizes on the server side. Do not rely on client-side validation alone, as it can be bypassed. Check the file MIME type and use PHP functions like getimagesize()
for images to ensure the file is what it claims to be.
Storing Files Securely
Store uploaded files outside the webroot when possible, or protect them with .htaccess rules. Rename files upon upload to avoid executable filenames or overwriting existing files.
Keeping Up with PHP Security Developments
Staying informed about the latest security developments in PHP is essential:
Subscribe to Security Newsletters and Forums
Follow PHP security blogs, newsletters, and forums. These resources can provide timely updates on vulnerabilities, patches, and best practices.
Participate in PHP Communities
Engage with PHP communities, such as PHP.net, Stack Overflow, and GitHub. These platforms can offer valuable insights and peer-reviewed solutions to common security challenges.
Regular Security Audits
Conduct regular security audits of your PHP codebase. Tools like PHPStan, Psalm, and security-focused linters can help identify potential vulnerabilities.
Advanced Security Features in PHP
Delving deeper into PHP’s capabilities reveals advanced features that can significantly enhance application security:
Securing File Inclusions
File inclusion vulnerabilities arise when using functions like include
or require
. To mitigate risks, avoid including files based on user input. Utilize __DIR__
and __FILE__
constants for safer file paths and consider implementing an allowlist of permissible files.
Open_basedir Restriction
Use PHP’s open_basedir
directive to limit the files that can be opened by PHP to a specified directory. This restriction prevents PHP scripts from accessing files outside the designated path, protecting against directory traversal attacks.
Disabling Dangerous Functions
PHP allows you to disable potentially dangerous functions via the disable_functions
directive in the php.ini
file. Functions like exec()
, shell_exec()
, and eval()
can be abused by attackers to execute arbitrary code.
Monitoring and Logging for Security
Effective monitoring and logging are vital for early detection of security incidents:
Implementing Robust Logging
PHP’s error logging capabilities should be leveraged to record suspicious activities. Ensure logs include sufficient details like timestamps, IP addresses, and specific error messages. Store logs securely and review them regularly.
Monitoring Tools
Use tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Graylog for comprehensive log management. These tools can aggregate logs from various sources, providing a centralized platform for analysis and alerting.
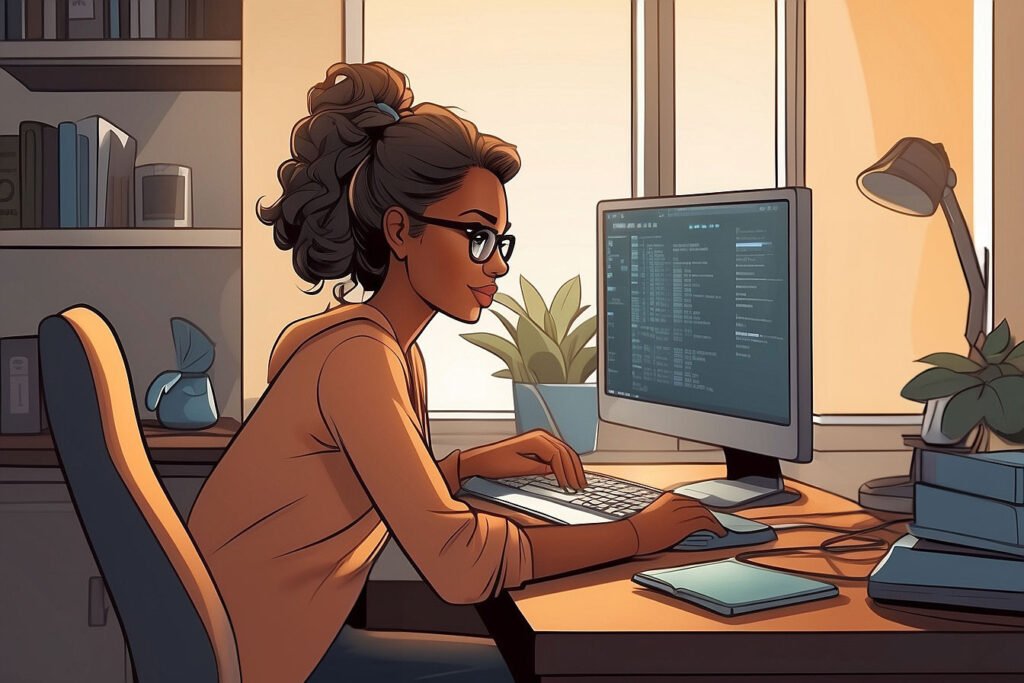
Handling Legacy PHP Code
Maintaining and securing legacy PHP code presents unique challenges:
Refactoring for Security
Legacy code often lacks modern security practices. Prioritize refactoring critical sections of the code, especially those handling authentication, user input, and database interactions.
Incremental Updates
Gradually update the legacy codebase to newer PHP versions. This may involve rewriting parts of the code to be compatible with modern PHP standards and security practices.
Third-Party Libraries and Frameworks
The use of third-party libraries and frameworks can impact security:
Vetting Dependencies
Carefully vet any third-party code before inclusion. Check the library’s or framework’s reputation, frequency of updates, and its security history.
Keeping Dependencies Updated
Regularly update third-party libraries and frameworks to their latest versions. Utilize tools like Composer for dependency management in PHP, and monitor for security updates.
Preparing for and Responding to Security Breaches
Even with robust security measures, preparing for potential breaches is essential:
Incident Response Plan
Develop a comprehensive incident response plan detailing steps to take in the event of a breach. This includes identifying the breach, containing the damage, eradicating the threat, and recovering affected systems.
Regular Security Drills
Conduct regular security drills to test the effectiveness of your response plan. Ensure that all team members know their roles and responsibilities during an incident.
Advanced Authentication Methods in PHP
Evolving cyber threats necessitate advanced authentication methods to safeguard user data and access:
Multi-Factor Authentication (MFA)
Implementing Multi-Factor Authentication adds an extra layer of security. This could involve a combination of something the user knows (password), something they have (a mobile device), and something they are (biometric verification).
OAuth and Social Logins
Integrating OAuth for authentication allows users to log in using their existing social media accounts, leveraging the security measures of established platforms. Ensure the implementation follows the latest OAuth 2.0 standards.
Encryption Techniques in PHP
Robust encryption is critical for protecting sensitive data:
Using Modern Encryption Libraries
Utilize modern PHP encryption libraries like OpenSSL or Sodium for strong encryption. These libraries offer functions for encrypting and decrypting data, generating cryptographic keys, and more.
Secure Key Management
Properly manage encryption keys by storing them securely, separate from the data they encrypt. Consider using a hardware security module (HSM) or a dedicated key management service.
PHP API Security Best Practices
Securing APIs is crucial in PHP applications, especially for those providing public APIs:
API Rate Limiting
Implement rate limiting to protect your API from brute force attacks and abuse. This can be achieved using server configurations or PHP libraries designed for API security.
JWT for Statelessness
Use JSON Web Tokens (JWT) for stateless authentication in APIs. JWTs allow for secure transmission of information between parties and are particularly useful in microservices architectures.
Security-First Culture in Development
Fostering a security-first mindset among developers is key to proactive security:
Regular Security Training
Conduct regular training sessions for developers on the latest security threats and mitigation techniques. This keeps the team updated and vigilant.
Code Reviews and Pair Programming
Implement code reviews and pair programming practices to catch security flaws early. Peer reviews are crucial for maintaining high-quality, secure code.
Continuous Security Auditing and Testing
Ongoing security assessments are vital for maintaining the integrity of your PHP application:
Automated Security Scanning
Use tools for automated security scanning of your PHP codebase. Static application security testing (SAST) and dynamic application security testing (DAST) tools can identify vulnerabilities before deployment.
Regular Penetration Testing
Engage in regular penetration testing conducted by external security experts. This simulates real-world attacks and identifies potential security weaknesses in your application.
Securing PHP E-Commerce Platforms
E-commerce platforms require additional security measures due to the sensitive nature of transactions and personal data involved:
Secure Payment Gateways
Integrate secure and reputable payment gateways. Ensure that payment processing is compliant with standards like PCI DSS (Payment Card Industry Data Security Standard) to protect customer payment information.
Regular Security Audits for E-Commerce
Conduct thorough security audits focusing on all aspects of the e-commerce workflow. This includes customer data handling, transaction processing, and interactions with third-party services.
Effective User Permission Management
Managing user permissions effectively is crucial for limiting the scope of potential breaches:
Role-Based Access Control (RBAC)
Implement RBAC to define roles and assign permissions based on those roles. This ensures users have access only to the resources necessary for their role, minimizing the risk of unauthorized access.
Regular Review of Access Rights
Regularly review and update user access rights, especially after role changes or departures. This prevents accumulation of unnecessary access privileges over time.
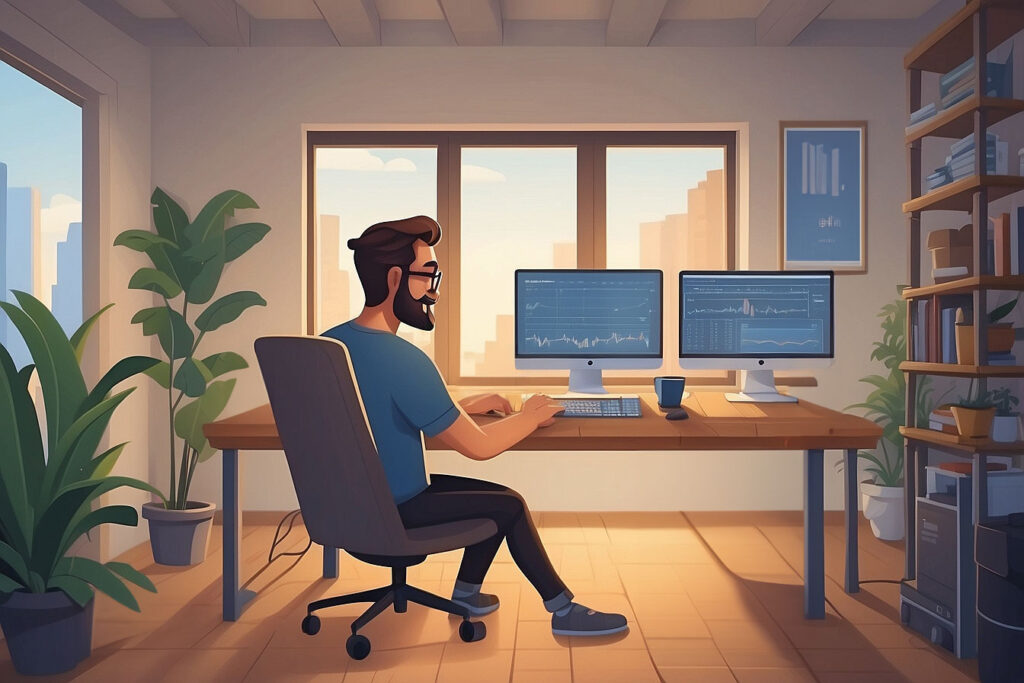
The Role of HTTPS in PHP Security
HTTPS plays a fundamental role in securing PHP applications:
Enforcing HTTPS
Enforce HTTPS on your PHP site to ensure all data transmitted between the client and server is encrypted. This is crucial for protecting sensitive information like login credentials and personal data.
HSTS (HTTP Strict Transport Security)
Implement HSTS to prevent protocol downgrade attacks and cookie hijacking. HSTS tells browsers to only interact with your server using secure HTTPS connections.
Balancing Performance and Security
While security is paramount, it’s important to balance it with application performance:
Efficient Security Practices
Implement security measures that do not significantly degrade performance. For example, use efficient hashing algorithms and optimize database queries for security features.
Caching for Performance
Use caching strategically to improve performance without compromising security. Be cautious with caching sensitive data and implement proper cache invalidation strategies.
Future Trends in PHP Security
Staying ahead in security involves being aware of emerging trends and future developments:
Embracing AI and Machine Learning
Look towards integrating AI and machine learning for advanced threat detection and automated security responses. These technologies can help identify and react to security threats more efficiently.
Preparing for Quantum Computing
Stay informed about the implications of quantum computing on cryptography. Future-proof your encryption methods to be resistant against potential quantum computing threats.
Security Considerations for PHP Frameworks
Selecting and utilizing PHP frameworks involves specific security considerations to bolster application safety:
Framework-Specific Security Features
Understand and leverage the built-in security features of your chosen PHP framework. Frameworks like Laravel, Symfony, and CodeIgniter offer features like CSRF protection, input validation, and ORM (Object-Relational Mapping) for secure database interactions.
Keeping Frameworks Updated
Regularly update your PHP framework to the latest version. Framework updates often include security patches and improvements that address newly discovered vulnerabilities.
Integrating CDN for Enhanced Security
Content Delivery Networks (CDNs) can significantly enhance the security of PHP applications:
DDoS Protection and Traffic Filtering
CDNs provide DDoS protection by distributing traffic across multiple servers. They also filter malicious traffic, reducing the risk of attacks reaching your server.
Secure Content Delivery
CDNs can ensure secure and encrypted content delivery, often offering SSL/TLS offloading, which can improve performance while maintaining security.
Logging and Monitoring Best Practices in PHP
Effective logging and monitoring are critical for detecting and responding to security incidents:
Comprehensive Logging Strategy
Develop a comprehensive logging strategy that includes error logs, access logs, and audit trails. Ensure logs are detailed enough to provide insight into potential security incidents.
Real-Time Monitoring Tools
Utilize real-time monitoring tools to detect and alert on unusual activities. Tools like Nagios, Zabbix, or cloud-based solutions can monitor server health, application performance, and security anomalies.
Secure Third-Party Integrations
Third-party integrations can introduce vulnerabilities if not handled securely:
Scrutinize Third-Party Services
Carefully evaluate third-party services for security practices before integration. Check for compliance with security standards and conduct regular security assessments.
Secure API Usage
When integrating external APIs, ensure secure communication channels like HTTPS. Manage API keys securely and monitor for unusual activities associated with API use.
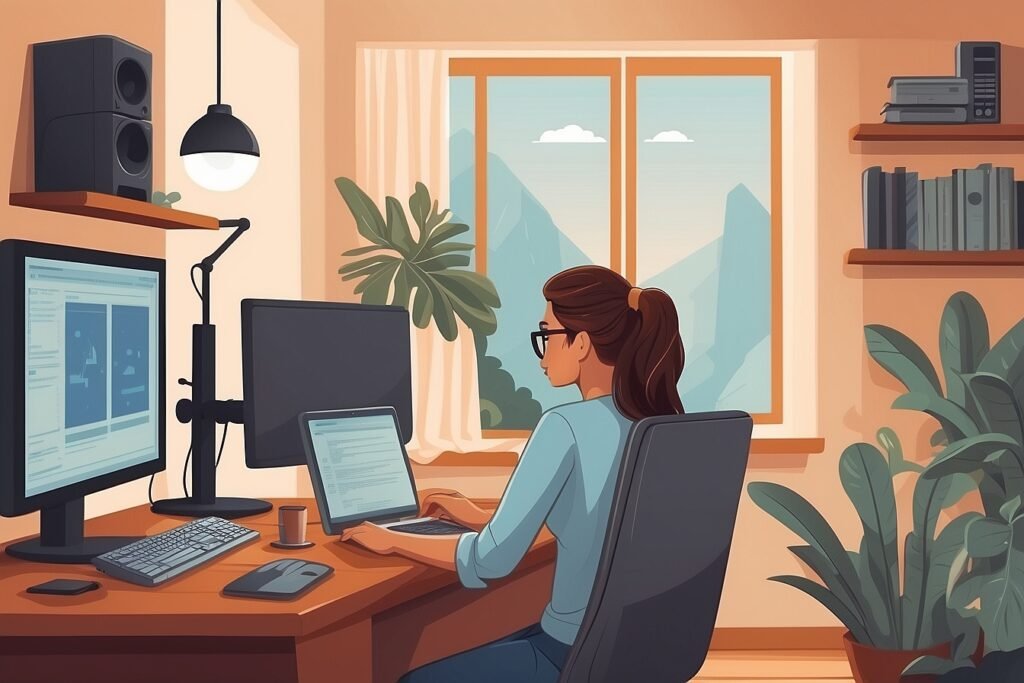
Compliance with Data Protection Regulations
Complying with global data protection regulations is crucial for PHP applications handling personal data:
Understanding GDPR and Other Regulations
Familiarize yourself with the General Data Protection Regulation (GDPR) and other relevant data protection laws. Ensure your PHP application adheres to these regulations in terms of user data collection, processing, and storage.
Implementing Privacy by Design
Adopt a ‘privacy by design’ approach, where data protection is integrated into the development process from the outset. This includes data minimization, encryption, and providing users with control over their data.
Table: PHP Web Application Security Checklist
Security Aspect | Key Actions | Tools/Techniques | Best Practices |
---|---|---|---|
Code Security | Validate and sanitize inputs, handle errors securely | htmlspecialchars() , Prepared Statements | Avoid revealing internal paths or details in error messages |
Authentication & Authorization | Implement robust login systems, use hashing for passwords | bcrypt, OAuth, MFA | Regularly update passwords and access rights |
Session & Cookie Management | Secure session handling, set cookie attributes | session_regenerate_id() , Secure & HttpOnly flags | Use timeouts and restrict session persistence |
Data Protection | Encrypt sensitive data, use SSL/TLS | OpenSSL, HTTPS, HSTS | Keep encryption methods up-to-date |
XSS Prevention | Encode output, implement CSP | htmlspecialchars() , Content Security Policy | Regularly review and update CSP rules |
CSRF Mitigation | Use anti-CSRF tokens, configure SameSite for cookies | CSRF Tokens, SameSite attribute | Validate tokens on each form submission |
Database Security | Use least privilege principle, audit databases | MySQLi, PDO | Regular backups and monitoring for unusual activities |
File Handling | Validate and securely store uploads | File MIME checking, storing outside webroot | Rename files upon upload, limit upload size |
Monitoring & Logging | Implement detailed logging, use real-time monitoring | ELK Stack, Graylog | Regularly review logs for anomalies |
Frameworks & Libraries | Use built-in features, keep updated | Laravel, Symfony, CodeIgniter | Regularly check for updates and patches |
API Security | Rate limit, use JWT for statelessness | Rate limiting, JSON Web Tokens | Monitor API usage and secure endpoints |
Compliance | Adhere to data protection laws like GDPR | Privacy by Design, Data Minimization | Regular legal reviews and updates |
Conclusion
Securing PHP web applications is an ongoing process requiring vigilance and continuous improvement. From implementing basic security principles like validating user input and encrypting data to adopting advanced strategies like multi-factor authentication and real-time monitoring, developers must stay abreast of the latest security trends and threats. Utilizing the strengths of PHP frameworks, integrating secure third-party services, and adhering to global data protection regulations are also critical. By systematically addressing each aspect of security, as outlined in the table above, PHP applications can be fortified against the evolving landscape of cyber threats.
Frequently Asked Questions
What is the most common security vulnerability in PHP applications?
The most common vulnerabilities in PHP applications include SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF). Regularly updating the PHP version and adhering to secure coding practices can mitigate these risks.
How can I protect my PHP application from SQL injection attacks?
Protect your PHP application from SQL injection by using prepared statements with PDO or MySQLi, validating and sanitizing user inputs, and employing the least privilege principle for database access.
Is it safe to use third-party libraries in my PHP application?
Third-party libraries can be safe if they are carefully vetted for security, regularly updated, and sourced from reputable providers. Always review the security practices and update history of any library before integrating it into your application.
How important is HTTPS for PHP web application security?
HTTPS is crucial for PHP web application security as it encrypts data transmitted between the client and server, protecting it from eavesdropping and tampering. Implementing HTTPS, along with HSTS, is essential for securing web applications.
Can PHP frameworks enhance the security of my application?
Yes, PHP frameworks like Laravel, Symfony, and CodeIgniter come with built-in security features such as CSRF protection, input validation, and secure authentication mechanisms. These features, combined with regular framework updates, can significantly enhance application security.
What steps should I take if my PHP application is breached?
In the event of a breach, follow your incident response plan: identify and contain the breach, eradicate the threat, recover affected systems, and notify affected parties. Conduct a thorough investigation to prevent future incidents and update your security measures accordingly.
How does GDPR impact PHP applications?
GDPR impacts PHP applications by imposing strict requirements on how personal data is collected, processed, and stored. Applications must ensure user consent, data minimization, and provide users with control over their data to comply with GDPR.
What is a Content Security Policy (CSP) and how does it help in PHP security?
A Content Security Policy (CSP) helps in PHP security by specifying which resources (like scripts, images, stylesheets) are allowed to load and execute in the browser, thereby preventing XSS attacks and other script-related vulnerabilities.